Creating your own simple webservice from java classes
- create a Java project in eclipse or whichever IDE you are using
- create following package webservicesTest.simplews
- Define two java classes Simple1.java and Simple2.java
- Simple1.java components addNumbers(a, b) and subtractNumbers(a,b)
- Simple2.java components multiplyNumbers(a, b) and divideNumbers(a, b)
- Create webservices.xml for defining the xsd and operations of above methods to expose
- Build web-services using ant tags as defined under build.xml (refer code)
- Use ANT tags for creating .ear and .war types to deploy webservice (refer code)
- Deploy the webservice to your weblogic or tomcat application server
- Redeploy your application server
- Invoke the WS methods using Dynamic (explained below refer WSClient.java)
Simple1.java
package webservicesTest.simplews;
public final class Simple1 {
public int addNumbers(int a, int b) {
System.out.println("a, b: "+a+", "+ b);
return (a+b);
}
public int subtractNumbers(int a, int b) {
System.out.println("a, b: "+a+", "+ b);
return (b-a);
}
}
Simple2.java
package webservicesTest.simplews;
public final class Simple2 {
public int multiplyNumbers(int a, int b) {
System.out.println("a, b: "+a+", "+ b);
return (a*b);
}
public int divideNumbers(int a, int b) {
System.out.println("a, b: "+a+", "+ b);
if(a==0) return 0;
return (b/a);
}
}
web-services.xml
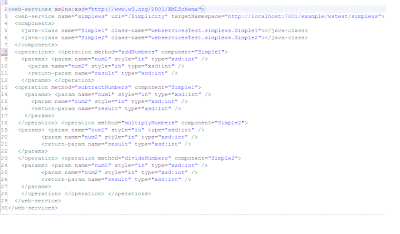
application.xml
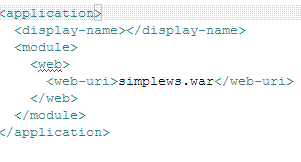
Build.xml
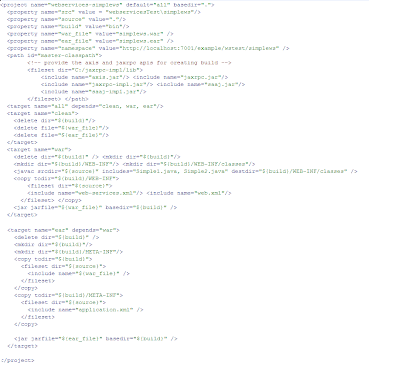
in the root of your project folder two files will be created on running above build.xml
simplews.ear and simplews.war
you can use any of the above files to deploy into your application server
after deploying the ws must be accessible using below uri
http://localhost:7001/simplews/Simplicity
WSDL
http://localhost:7001/simplews/Simplicity?wsdl
Now access your webservice and invoke it's methods from a java client
This is dynamic invocation where you donot need to make a client or stub for invoking ws methods and can do directly using the http url.
WSClient.java
package webservicesTest.simplews;
import javax.xml.rpc.Call;
import javax.xml.rpc.ParameterMode;
import javax.xml.rpc.ServiceFactory;
import javax.xml.rpc.Service;
import javax.xml.namespace.QName;
public final class WSClient {
private static String TARGET_NAMESPACE =
"http://localhost:7001/example/wstest/simplews";
public static void main(String[] argv) throws Exception {
// Setup the global JAX-RPC service factory
System.setProperty( "javax.xml.rpc.ServiceFactory",
"weblogic.webservice.core.rpc.ServiceFactoryImpl");
String url = "http://localhost:7001//simplews/Simplicity";
System.out.println("Calling Web Service at: "+url);
System.out.println("");
//create service factory
ServiceFactory factory = ServiceFactory.newInstance();
QName serviceName = new QName(TARGET_NAMESPACE, "simplews");
QName portName = new QName(TARGET_NAMESPACE, "simplewsPort");
//create service
Service service = factory.createService(serviceName);
//create call
Call subtraction = service.createCall();
Call multiplication = service.createCall();
//set port and operation name
subtraction.setPortTypeName(portName);
subtraction.setOperationName(new QName("subtractNumbers"));
multiplication.setPortTypeName(portName);
multiplication.setOperationName(new QName("multiplyNumbers"));
//add parameters
subtraction.addParameter("num1",
new QName("http://www.w3.org/2001/XMLSchema", "int"),
ParameterMode.IN);
subtraction.addParameter("num2",
new QName("http://www.w3.org/2001/XMLSchema", "int"),
ParameterMode.IN);
subtraction.setReturnType(new QName( "http://www.w3.org/2001/XMLSchema","int") );
multiplication.addParameter("num1",
new QName("http://www.w3.org/2001/XMLSchema", "int"),
ParameterMode.IN);
multiplication.addParameter("num2",
new QName("http://www.w3.org/2001/XMLSchema", "int"),
ParameterMode.IN);
multiplication.setReturnType(new QName( "http://www.w3.org/2001/XMLSchema","int") );
//set end point address
subtraction.setTargetEndpointAddress(url);
multiplication.setTargetEndpointAddress(url);
Integer x1 = (Integer) subtraction.invoke(
new Object [] { new Integer(123), new Integer(10) }
);
System.out.println("** subtraction returned: "+x1);
Integer x2 = (Integer) multiplication.invoke(
new Object [] { new Integer(123), new Integer(10) }
);
System.out.println("** multiplication returned: "+x2);
}
}
No comments:
Post a Comment